To scrap the data from website, Website must be public and open for scrapable.
In the blow code, just update the CURLOPT_URL to which websites data you want to scrap.
In the blow code, just update the CURLOPT_URL to which websites data you want to scrap.
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "http://www.web-technology-experts-notes.in/");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_HEADER, 0);
$output = curl_exec($ch);
curl_close($ch);
echo $output;
• $message is used to store variable data. $$message can be used to store variable of a variable. Data stored in $message is fixed while data stored in $$message can be changed dynamically.
Example:
Example:
$var1 = ‘Variable 1’
$$var1= ‘variable2’
This can be interpreted as $ Variable 1=‘variable2’;
For me to print value of both variables, I will write
$var1 $($var1)
• $message is a variable and $$message is a variable of another variable.
Example
Example
$Message = "YOU";
$you= "Me";
echo $message //Output:- you
echo $$message //output :-Me
$$message allows the developer to change the name of the variable dynamically.
Urlencode can be used to encode a string that can be used in a url. It encodes the same way as posted data from web page is encoded. It returns the encoded string.
Syntax:
Syntax:
urlencode (string $str )
urlencode () is the function that can be used conveniently to encode a string before using in a query part of a URL. This is a convenient way for passing variables to the next page.
Syntax:
Syntax:
urldecode (string $str )
urldecode() is the function that is used to decode the encoded string. Urldecode can be used to decode a string. Decodes any %## encoding in the given string (Inserted by urlencode.)
header(‘Content-Type: text/html; charset=utf-8′);
Xdebug: – It uses the DBGp debugging protocol for debugging.
The debug information that Xdebug can provide includes the following:
• stack and function traces in error messages with:
• full parameter display for user defined functions
• function name, file name and line indications
• support for member functions
• memory allocation
• protection for infinite recursions
Xdebug also provides:
• profiling information for PHP scripts
• code coverage analysis
• Capabilities to debug your scripts interactively with a debugger front-end.[4]
Xdebug is also available via PECL
The debug information that Xdebug can provide includes the following:
• stack and function traces in error messages with:
• full parameter display for user defined functions
• function name, file name and line indications
• support for member functions
• memory allocation
• protection for infinite recursions
Xdebug also provides:
• profiling information for PHP scripts
• code coverage analysis
• Capabilities to debug your scripts interactively with a debugger front-end.[4]
Xdebug is also available via PECL
call_user_func(function() { echo ‘anonymous function called.'; });
There are different methods through which we can send mails in PHP. They are as follows:
1. PHP mail() function
It implicitly sends a message to SMTP server, which is configured in the php.ini file. This function is used by the base class of MIME message composing and sending package.
2. SMTP server relay
They are used to relay the messages to an intermediate SMTP server. This server stores the messages temporarily and will try to deliver them in the destination SMTP server.
3. Sending urgent messages by doing direct delivery to the destination SMTP server
A variable named direct_delivery is provided by the smtp_message_class sub-class, which connects to the destination SMTP server and sends the message directly.
1. PHP mail() function
It implicitly sends a message to SMTP server, which is configured in the php.ini file. This function is used by the base class of MIME message composing and sending package.
2. SMTP server relay
They are used to relay the messages to an intermediate SMTP server. This server stores the messages temporarily and will try to deliver them in the destination SMTP server.
3. Sending urgent messages by doing direct delivery to the destination SMTP server
A variable named direct_delivery is provided by the smtp_message_class sub-class, which connects to the destination SMTP server and sends the message directly.
– Include Net/DNS.php file in the beginning of the script
– Create an object for DNS resolver by using $ndr = Net_DNS_Resolver()
– Query the ip address using $ndr->search(“somesite.com”,”A”) and assign to a relevant variable. Ex: $result
– Now, display the value of $result
– Create an object for DNS resolver by using $ndr = Net_DNS_Resolver()
– Query the ip address using $ndr->search(“somesite.com”,”A”) and assign to a relevant variable. Ex: $result
– Now, display the value of $result
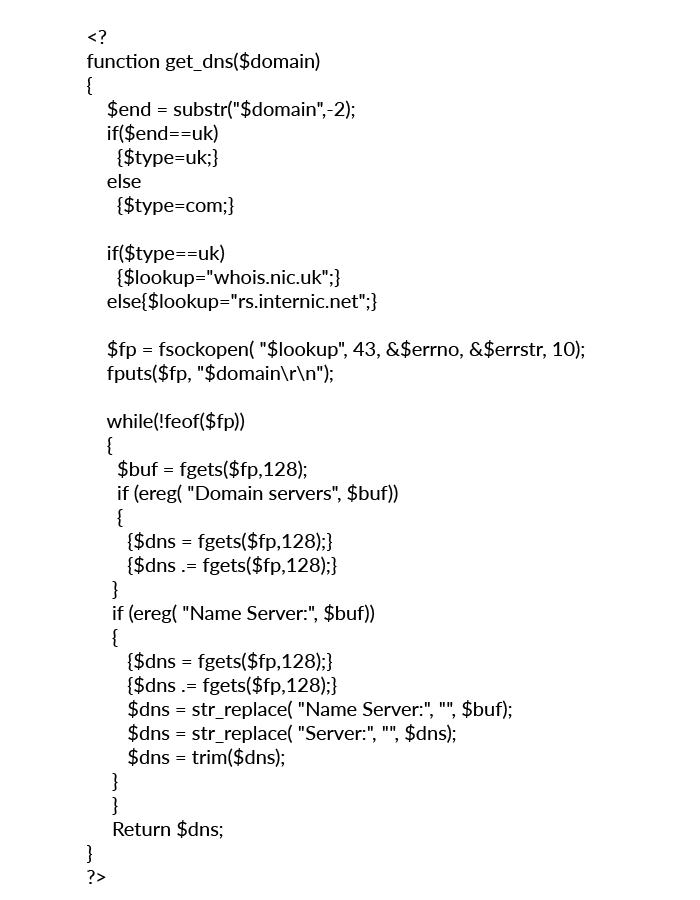
$startTime= microtime(true);
/** Write here you code to check **/
/** Write here you code to check **/
$endTime= microtime(true);
echo ‘Time Taken to execute the code:’.$endTime-$startTime
/** Write here you code to check **/
/** Write here you code to check **/
$endTime= microtime(true);
echo ‘Time Taken to execute the code:’.$endTime-$startTime
We can resolve these errors through php.ini file or through .htaccess file.
1. From php.ini file, increase the max_execution_time =360 (or more according to need)
and change memory_limit =128M (or more according to need)
2. From php file, we can increase time by writing ini_set(‘max_execution_time’,360 ) at top of php page to increase the execution time.And to change memory_limit write ini_set(‘memory_limit ,128M )
3. From .htaccess file, we can increase time and memory by:
1. From php.ini file, increase the max_execution_time =360 (or more according to need)
and change memory_limit =128M (or more according to need)
2. From php file, we can increase time by writing ini_set(‘max_execution_time’,360 ) at top of php page to increase the execution time.And to change memory_limit write ini_set(‘memory_limit ,128M )
3. From .htaccess file, we can increase time and memory by:
We can retrieve data in the result set of MySQL using PHP in four Ways
1. mysqli_fetch_row >> Get a result row as an enumerated array
2. mysqli_fetch_array >> Fetch a result row as associative and numeric array
3.mysqli_fetch_object >> Returns the current row of a result set as an object
4. mysqli_fetch_assoc >> Fetch a result row as an associative array
mysqli_fetch_object() is similar to mysqli_fetch_array(), with one difference –
an object is returned instead of an array, which implies that that we can only access the data by the field names, and not by their offsets (numbers are illegal property names).
1. mysqli_fetch_row >> Get a result row as an enumerated array
2. mysqli_fetch_array >> Fetch a result row as associative and numeric array
3.mysqli_fetch_object >> Returns the current row of a result set as an object
4. mysqli_fetch_assoc >> Fetch a result row as an associative array
mysqli_fetch_object() is similar to mysqli_fetch_array(), with one difference –
an object is returned instead of an array, which implies that that we can only access the data by the field names, and not by their offsets (numbers are illegal property names).
How can we destroy a session in PHP
Yes, we can include (“xyz.php”) more than one time in any page. But it creates a problem when a xyz.php file contains some function declaration- an error occurs due to an already present function in this file. Otherwise, there is no problem, for instance if you want to show same content two times in the page then you must include it two times.
Yes, we can include (“xyz.php”) more than one time in any page. But it creates a problem when a xyz.php file contains some function declaration- an error occurs due to an already present function in this file. Otherwise, there is no problem, for instance if you want to show same content two times in the page then you must include it two times.
mysqladmin -u root -p password “newpassword”
Once the Web server received the uploaded file, it will call the PHP script specified in the form action attribute to process them. This received PHP script can get the uploaded file information through a predefined array called $_FILES. Uploaded file information is organized in $_FILES as a two-dimensional array as:
• $_FILES[$fieldName][‘name’] : Original file name on the browser system.
• $_FILES[$fieldName][‘type’] : the file type determined by the browser.
• $_FILES[$fieldName][‘size’] : Number of bytes of the file content.
• $_FILES[$fieldName][‘tmp_name’] : a temporary filename of the file in which the uploaded file was stored on the server.
• $_FILES[$fieldName][‘error’] an error code associated with this file upload.
• The $fieldName is the name used in the <INPUT,>.
• $_FILES[$fieldName][‘name’] : Original file name on the browser system.
• $_FILES[$fieldName][‘type’] : the file type determined by the browser.
• $_FILES[$fieldName][‘size’] : Number of bytes of the file content.
• $_FILES[$fieldName][‘tmp_name’] : a temporary filename of the file in which the uploaded file was stored on the server.
• $_FILES[$fieldName][‘error’] an error code associated with this file upload.
• The $fieldName is the name used in the <INPUT,>.
If you want to include special characters like spaces in the query string, you need to protect them by applying the urlencode() translation function. The script below shows how to use urlencode():
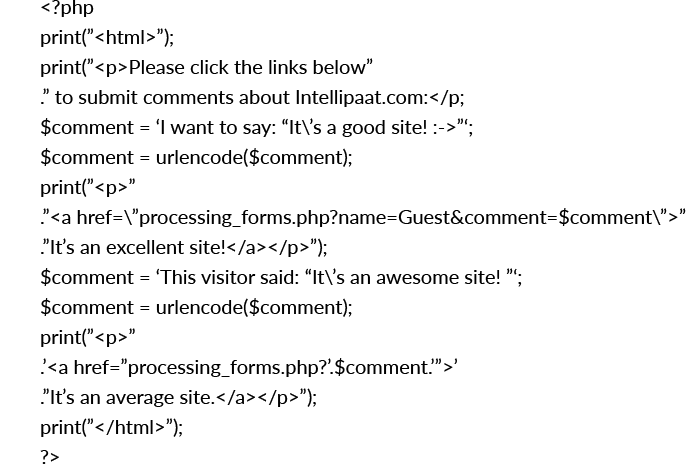
We can destroy a session by:

To delete a specific session variable, we use

function changevalue(&$y) { $y = $y + 7; } $num = 8;
changevalue($num);
echo $num;
I really enjoy the blog article.Much thanks again.
ReplyDeleteOSB training
OTM online training
OTM training
SAS online training
SAS training
structs online training
structs training
Webmethods online training
Webmethods training
Wise package studio online training
Wise package studio training
Hey, thanks for the blog article.Really looking forward to read more. Cool.
ReplyDeletePython Django online training
Python Django training
Python online training
Python training
R programming online training
R programming training
Salesforce admin online training
Salesforce admin training
Digital marketing is the component of marketing that utilizes internet and online based digital technologies such as desktop computers, mobile phones and other digital media and platforms to promote products and services.
ReplyDeleteDigital Marketing Training in Hyderabad
Digital Marketing Course in Hyderabad
Java applications are typically compiled to bytecode that can run on any Java virtual machine (JVM) regardless of the underlying computer architecture. The syntax of Java is similar to C and C++, but has fewer low-level facilities than either of them.
ReplyDeleteJava Training in Hyderabad
Java Course in Hyderabad
Data science Training in Hyderabad. Data science is an inter-disciplinary field that uses scientific methods, processes, algorithms and systems to extract knowledge and insights from many structural and unstructured data. Data science is related to data mining, machine learning and big data.
ReplyDeleteData Science Training In Hyderabad
Data Science Course In Hyderabad
Great
ReplyDeleteVery Helpful
Thanks For Sharing Such A Wonderful Blog
For Data Science Course Data Science course in Bangalore
Great very helpful blog. Thanks For Sharing Such A Wonderful Blog. I will definitely go ahead and take Advantage of this. Your Blog Is Very Informative. Again Thanks For Sharing This Blogs With Us. For more learning go through skillslash.
ReplyDeleteFor Data Science Course Data Science course in Bangalore
Best College for Commerce students
ReplyDeleteca colleges in hyderabad
mec inter colleges in hyderabad
cec inter colleges in hyderabad
Cma Colleges in hyderabad
degree colleges in hyderabad
cs colleges in hyderabad
Great Article Artificial Intelligence Projects
ReplyDeleteProject Center in Chennai
JavaScript Training in Chennai
JavaScript Training in Chennai Project Centers in Chennai
Very Useful article, Thanks For Sharing With Us
ReplyDeleteHere a related Stuff:
Adf Training In Hyderabad
Adf Online Training
Adf Training In Ameerpet
Adf Online Training